Boolean Games Solver
Content and Instructions
This webpage contains the data, source code and instructions for the Boolean Games Solver, developed by Kim Bauters and Sofie De Clercq.
The solver is equipped to compute pure Nash equilibria (PNEs) or core elements of Boolean games (BGs). The BGs are allowed to include costs and constraints. Additionally, the solver is able to search for Pareto optimal equilibria. References to accompanying scientific papers are:
- De Clercq, S.; Bauters, K.; Schockaert, S.; Mihaylov, M.; De Cock, M.; and Nowé, A. 2014.
Decentralized computation of Pareto optimal pure Nash equilibria of Boolean games with privacy concerns.
Proc. ICAART '14
[PDF]
- De Clercq, S.; Bauters, K.; Schockaert, S.; De Cock, M.; and Nowé, A. 2014.
Using Answer Set Programming for Solving Boolean Games.
Proc. KR '14
[PDF]
- De Clercq, S.; Bauters, K.; Schockaert, S.; Mihaylov, M.; De Cock, M.; and Nowé, A. 2014. Exact and Heuristic Methods for Solving Boolean Games. To be submitted.
Suppose G is a BG with 3 agents. Agent 1 controls one action variable a, agent 2 controls one action variable b, agent 3 controls two action variables c and d.
The goal of agent 1 is a ⇔ c, the goal of agent 2 is b ∨ (a ∧ ¬d) and the goal of agent 3 is (c ∨ a) ⇒ b.
Agent 3 is constraint by ¬(c ∧ d). Undertaking action a involves a cost of 3 to agent 1. Not undertaking it involves a cost of 1.
# this is a comment line
# we describe the BG G
# the unique game line starts with a 'p', followed by the type of game ('bg'), followed by the number of agents n
p bg 3
# all agents are numbered from 1 untill n
# for each agent, a line starting with 'a' specifies the action variables the agent controls
# this a-line always ends with a zero
a 1 a 0
a 2 b 0
a 3 c d 0
# the goals are specified in a line starting with the agent id nr
# for each agent a goal must be specified
1 a <=> c
2 b ; (a & -d)
3 (c ; a) => b
# costs and constraints can be added optionally
# a constraint starts with an 's', followed by the agent id nr and the constraint
s 3 -(c & d)
# a cost starts with a 'c', followed by the action variable
# first the cost for undertaking the action is given
# then the cost for not undertaking the action
# the implementation only allows integers
c a 3 1
# as soon as one action variable has a cost line, all action variables without cost lines are interpreted as involving a cost of zero for undertaking the action or not.
If your BG does not include costs or constraints, you can respectively leave out the c-lines or the s-lines.
The source code folder contains several programs. To use them, copy and unzip the source code folder and change the terminal direction to this folder. Then follow the instructions below to compile the programs. Once compiled, you can get the usage instructions of the programs by either executing them without options or arguments, or by adding the option ' -h' to the command.
Boolean Game Generators
- generatedagbg
This is a BG generator, generating BGs such that the irreflexive part of the dependency graph is acyclic. It has multiple parameters:- -a : the number of agents
- -b : the number of action variables per agent
- -m : the maximum number of operators in the goal of an agent
- -c : the probability of a binary operator being a conjunction (as opposed to a disjunction or negation) [default: 0.33]
- -d : the probability of a binary operator being a disjunction (as opposed to a conjunction or negation) [default: 0.33]
- -e : the probability that agent i depends on agent j (j ≥ i)
- -s : the seed [default: 1]
Compile this generator with:
g++ generatedagbg.cc command_line_options.cc atom.cc asphelper.cc -O3 -o generatedagbg
example of execution:
./generatedagbg -a 4 -b 2 -m 2 -c 0.33 -d 0.33 -e 0.7 -s 10
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 4
a 1 p1 p2 0
a 2 p3 p4 0
a 3 p5 p6 0
a 4 p7 p8 0
1 (p4 ; p5)
2 -p3
3 -p8
4 (p7 ; p8)
- generatebgforasp
This is a BG generator, generating random BGs. It has multiple parameters:- -a : the number of agents
- -b : the number of action variables per agent
- -m : the maximum number of binary operators in the goal of an agent
- -c : the probability of a binary operator being a conjunction (as opposed to a disjunction)
- -n : the probability of an atom appearing in a goal being negated
- -o : make sure that agent's goal contains at least one of his own action variables [default: 0]
- -s : the seed [default: 1]
Compile this generator with:
g++ generatebgforasp.cc command_line_options.cc propparser.cc proposition.cc atom.cc asphelper.cc -O3 -o generatebgforasp
example of execution:
./generatebgforasp -a 5 -b 3 -m 4 -c 0.4 -n 0.6 -o 1 -s 7
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 5
a 1 p1 p2 p3 0
a 2 p4 p5 p6 0
a 3 p7 p8 p9 0
a 4 p10 p11 p12 0
a 5 p13 p14 p15 0
1 ((-p8 & -p14) ; p2)
2 (p10 ; ((-p1 ; p6) & -p4))
3 (((-p1 ; p9) & p13) ; -p9)
4 (-p14 ; (p6 & -p10))
5 (-p6 & (-p13 & (p7 ; p14)))
- generateprojectbg
This is a BG generator, generating project BGs (see generateprojectbg.cc for more info). It has multiple parameters:- -a : the number of agents
- -p : the probability that a random agent is a partner as well as the probability that a random agent, in case it's not a partner, is an anti-partner
- -n : the number of projects
- -c : add costs and constraints [default: 0]
- -s : the seed [default: 1]
i per project m. Setting it to true means joining the project. For every project, every agent is randomly assigned one of 13 types, which are determined by three parameters:- personal preference: (a) join the project, (b) do not join, and (c) no preference;
- positive partnership (only relevant if level 1 is not (b)): (a) no condition, (b) only join a project if at least one partner joins the project, and (c) only join a project if all partners join the project;
- negative partnership (only relevant if level 1 is not (b)): (a) no condition, and (b) only join the project if no anti- partners join the project.
i ∧ ((pmj ∨ pmk ) ∧ ¬pml )) ∨ ¬pmi . So either agent i joins the project with j or k and without l, or agent i does not join. The overall goal of an agent is formed as the conjunction of the sub-goals corresponding to all the projects.
compile with:
g++ generateprojectbg.cc command_line_options.cc -O3 -o generateprojectbg
example of execution:
./generateprojectbg -a 4 -p 0.6 -n 1 -s 7
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 4
a 1 p1_1 0
a 2 p2_1 0
a 3 p3_1 0
a 4 p4_1 0
1 -p1_1
2 ((p2_1 & (p1_1 ; p4_1)) ; -p2_1)
3 -p3_1
4 ((p4_1 & -p2_1) ; -p4_1)
- generatebg
This is a BG generator, generating random BGs which have at least one strategy profile such that every agent reaches its goal. It has multiple parameters:- -a : the number of agents
- -b : the number of action variables per agent
- -m : the maximum number of operators in the goal of an agent
- -c : the probability of an operator being a conjunction (as opposed to a disjunction or a negation)
- -d : the probability of an operator being a disjunction (as opposed to a conjunction or a negation)
- -s : the seed [default: 1]
compile with:
g++ generatebg.cc command_line_options.cc propparser.cc proposition.cc atom.cc asphelper.cc -O3 -o generatebg
example of execution:
./generatebg -a 4 -b 2 -m 4 -c 0.33 -d 0.33 -s 6
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 4
a 1 p1 p2 0
a 2 p3 p4 0
a 3 p5 p6 0
a 4 p7 p8 0
1 (p2 ; -(p6 & p7))
2 -(p5 & p3)
3 -(p2 ; p3)
4 -(p4 ; p1)
- generatebgneglit2
This is a BG generator, generating random BGs which have at least one strategy profile such that every agent reaches its goal. The generated goals are in negation normal form. The generator has multiple parameters:- -a : the number of agents
- -b : the number of action variables per agent
- -c : the number of conjuncts in each goal
- -d : the number of disjuncts in each goal
- -m : c and d interpreted as maximum as opposed to fixed [default: 0]
- -n : the probability of negations appearing in the goals
- -s : the seed [default: 1]
compile with:
g++ generatebgneglit2.cc command_line_options.cc propparser.cc proposition.cc atom.cc asphelper.cc -O3 -o generatebgneglit2
example of execution:
./generatebgneglit2 -a 4 -b 2 -c 2 -d 2 -m 0 -n 0.5 -s 7
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 4
a 1 p1 p2 0
a 2 p3 p4 0
a 3 p5 p6 0
a 4 p7 p8 0
1 (p6 ; (-p4 & (p6 & p7)))
2 (p1 & (-p3 & (-p8 ; -p5)))
3 (p5 & ((-p1 & p4) ; p4))
4 (-p2 ; ((p5 & p6) & p6))
- generatewsnbg
This is a BG generator, generating BGs corresponding to wireless sensor networks (WSNs), based on 'Decentralized Reinforcement Learning for Energy-Efficient Scheduling in Wireless Sensor Network' by M. Mihaylov. The generator has multiple parameters:- -a : parameter inferring the number of agents
- -t : the topology (1=line, 2=grid, 3=highly connected grid, 4=mesh)
- -s : the seed [default: 1]
In a WSN, sensor nodes are ordered in a network. Connected sensor can exchange information. Some of the sensors are connected to the sink. Generally, the goal of each sensor is to send its information to the sink, through its neighbors, without other sensors interferring this transmission. There are 4 possible topologies of the WSN:- line-topology
- grid-topology
- highly connected grid-topology
- mesh-topology
compile with:
g++ generatewsnbg.cc command_line_options.cc -O3 -o generatewsnbg
example of execution:
./generatewsnbg -a 2 -t 3
corresponding output (might not be exactly the same, because of difference in 'seed' function on different devices):
p bg 4
a 1 p_1 0
a 2 p_2 0
a 3 p_3 0
a 4 p_4 0
1 (p_1 & (p_3 ; p_4) & -p_2) ; (-p_1 & (p_2))
2 (p_2 & (p_3 ; p_4) & -p_1) ; (-p_2 & (p_1))
3 (p_3 & (p_1 ; p_2) & -p_4) ; -p_3
4 (p_4 & (p_1 ; p_2) & -p_3) ; -p_4
Boolean Game Methods
- bg2wslps and bg2wslpscon
These are two iterative stochastic algorithms WSLpSsim and WSLpScon, designed for BGs with a solution for which every agent reaches its goal (i.e. for these BGs convergence is theoretically guaranteed without checking whether an equilibrium is reached). The goal of the algorithms is to find a Pareto optimal PNE.
In the algorithm WSLpSsim all agents simultaneously switch actions in each iteration; in the algorithm WSLpScon the agents consecutively switch action (one agent per iteration, in a fixed order). Benchmarks are ran with a BG generator (either 'generatewsnbg' or 'generatebgneglit2', depending on the specified parameters). Alternatively, it can also be used on a specific BG, using a file as input. These algorithms have multiple parameters:
Algorithm related options:
- -f : the file which contains the BG (e.g. output of a BG generator)
- -i : the maximum number of iterations [default: 1000]
- -n : the number of neighbors to ask whether to goal is satisfied [default: 1]
- -a : probability of involving all neighbors [default: 0]
- -l : alpha = the probability to shift (see paper [1])
- -q : compute alpha instead of using -l [default: 0]
- -s : timeout per game (in ms) [default: no timeout]
- -r : the number of runs per Boolean game [default: 1]
- -b : the number of benchmark runs
- -p : the number of agents
- -t : the topology (only for WSN-BGs)
- -v : the number of action variables per agent
- -c : the number of conjuncts (for random-BGs)
- -d : the number of disjuncts (for random-BGs)
- -g : probability of an atom in the goal being negated (for random-BGs)
- -m : c and d interpreted as maxima as opposed to fixed [default: 0] (for random-BGs)
compile respectively with:
g++ bg2wslps.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc wslps.cc timer.cc command_line_options.cc -O3 -o bg2wslps
g++ bg2wslpscon.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc wslpscon.cc timer.cc command_line_options.cc -O3 -o bg2wslpscon
This decentralized method supports BGs with costs and constraints, with a solution such that every agent reaches its goal.
- bg2wslpsbr and bg2wslpsbrcon
These are two iterative stochastic algorithms WSLpS-BRsim and WSLpS-BRcon, designed for general BGs. The difference with WSLpSsim and WSLpScon is that agents now ask their neighbors whether they play a best response instead of whether their goal is reached. The goal of the algorithms is to find a PNE.
In the algorithm WSLpS-BRsim all agents simultaneously switch actions in each iteration; in the algorithm WSLpS-BRcon the agents consecutively switch action (one agent per iteration, in a fixed order). Benchmarks are ran with a BG generator (either 'generatewsnbg' or 'generatebgneglit2', depending on the specified parameters). Alternatively, it can also be used on a specific BG, using a file as input. These algorithms have multiple parameters:
Algorithm related options:
- -f : the file which contains the BG (e.g. output of a BG generator)
- -i : the maximum number of iterations [default: 1000]
- -n : the number of neighbors to ask whether to goal is satisfied [default: 1]
- -a : probability of involving all neighbors [default: 0]
- -l : alpha = the probability to shift (see paper [1])
- -q : compute alpha instead of using -l [default: 0]
- -s : timeout per game (in ms) [default: no timeout]
- -r : the number of runs per Boolean game [default: 1]
- -b : the number of benchmark runs
- -p : the number of agents
- -t : the number of action variables per agent (for random- or DAGBGs)
- -m : the maximum number of operators per goal (for random- or DAGBGs)
- -c : the number of conjunctions (for random- or DAGBGs)
- -d : the number of disjunctions (only for DAGBGs)
- -j : the probability that agent i depends on agent j (j ≥ i) (for DAGBGs)
- -g : the probability of an atom in the goal being negated (only for random-BGs)
- -o : make sure own goal contains at least 1 own action variable [default: 0] (only for randomBG)
- -u : the probability that a random agent is a (anti-)partner (only for projectBG)
- -v : the number of projects [default: 1] (only for projectBGs)
- -z : add costs and constraints [default: 0] (only for projectBGs)
compile respectively with:
g++ bg2wslpsbr.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc wslpsbr.cc timer.cc command_line_options.cc -O3 -o bg2wslpsbr
g++ bg2wslpsbrcon.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc wslpsbrcon.cc timer.cc command_line_options.cc -O3 -o bg2wslpsbrcon
This decentralized method supports BGs with costs and constraints.
- bg2wslpspne
This is the same iterative stochastic algorithm as 'bg2wslps', but in addition, it is checked centrally whether a PNE or core element is reached. Therefore, it can be used on all Boolean games, not just those with a solution such that every agent reaches its goal. Benchmarks are ran with a BG generator (either 'generateprojectbg' or 'generatebgforasp', depending on the specified parameters). Alternatively, it can also be used on a specific BG, using a file as input. Its parameters are:
Algorithm related options:
- -f : the file specifying the Boolean game (overrides -b)
- -i : the maximum number of iterations [default: 1000]
- -n : the number of neighbors to ask whether to goal is satisfied [default: 1]
- -a : probability of involving all neighbors [default: 0]
- -e : search for PNEs (as opposed to core elements) [default: 1]
- -s : timeout per game (in ms) [default: no timeout]
- -r : the number of WSLpS-runs per Boolean game [default: 1]
- -b : specify the number of benchmark runs
- -p : the number of agents
- -t : the number of action variables per agent (only for random- or dagBG)
- -m : the maximum number of binary operators in the goal of an agent (only for random- or dagBG)
- -c : the probability of conjunctions (only for random- or dagBG)
- -d : the probability of disjunctions (only for dagBG)
- -j : the probability that agent i dependent of agent j (j≥ i) (for dagBG)
- -g : the probability of an atom appearing in a goal being negated (only for randomBG)
- -o : make sure that agent's goal contains at least one of his own action variables [default: 0] (only for randomBG)
- -u : the probability that a random agent is a partner as well as the probability that a random agent, in case it's not a partner, is an anti-partner (only for projectBG)
- -v : the number of projects (only for projectBG)
compile with:
g++ bg2wslpspne.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc wslps.cc timer.cc command_line_options.cc -O3 -o bg2wslpspne
This centralized method supports BGs with costs or constraints.
- bg2asp
This is a BG solver, based on disjunctive ASP. It can compute PNEs and core elements of BGs. Additionally, it can enforce Pareto optimality. Benchmarks are ran with a BG generator (either 'generateprojectbg', 'generatebgforasp' or 'generatedagbg', depending on the specified parameters). Alternatively, it can also be used on a specific BG, using a file as input. Its parameters are:
Algorithm related options:
- -i : [FLAG] only output the simulation; do not execute
- -f : the file specifying the Boolean game
- -n : the number of models to compute [default: all]
- -s : the ms before the ASP solver is abrupted [default: no timeout]
- -p : additionally demand Pareto optimality [default: 0]
- -e : search PNEs, as opposed to core elements [default: 1]
- -r : use clingo (0), DLV (1) or WASP (2) [default: 0]
- -b : the number of benchmark runs (1 run per game) (overrides -f)
- -a : the number of agents
- -t : the number of actions per agent (only for randomBG
- -m : the maximum number of operators per goal (only for randomBG)
- -c : the probability of conjunctions (only for randomBG)
- -d : the probability of disjunctions (only for dagBG)
- -j : the probability that agent i depends on agent j (j ≥ i) (only for DAGBGs)
- -g : the probability of negations (only for randomBG)
- -o : own goal contains at least 1 own action variable [default: 0] (only for randomBG)
- -u : the probability that a random agent is a (anti-)partner (only for projectBG)
- -v : the number of projects [default: 1] (only for projectBG)
- -z : add costs and constraints [default: 0] (only for projectBG)
compile with:
g++ bg2asp.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc timer.cc command_line_options.cc -O3 -o bg2asp
This centralized method supports BGs with costs or constraints.
Please note that this program uses an ASP-solver. Depending on the parameter value of , it uses either DLV or clingo. Therefore, to use this method, you also need to install DLV or clingo, which are freely available at respectively http://www.dlvsystem.com/dlv/ and http://potassco.sourceforge.net. As shown in paper [3], clingo is faster for larger problem instances.
- bg2aspnormal
This is a BG solver computing PNEs, based on an ASP encoding of De Vos and Vermeir (1999) for normal form games. Benchmarks are ran with a BG generator (either 'generateprojectbg', 'generatebgforasp' or 'generatedagbg', depending on the specified parameters). It has multiple parameters:
Algorithm related options:
- -i : [FLAG] only output the simulation; do not execute
- -f : the file specifying the Boolean game
- -n : the number of models to compute
- -s : the ms before the ASP solver is abrupted
- -b : the number of benchmark runs (1 run per game) (overrides -f)
- -a : the number of agents
- -t : the number of actions per agent (only for randomBG)
- -m : the maximum number of operators per goal (only for randomBG)
- -c : the probability of conjunctions (only for randomBG)
- -d : the probability of disjunctions (only for dagBG)
- -j : the probability that agent i depends on agent j (j ≥ i) (only for DAGBG)
- -g : the probability of negations (only for randomBG)
- -o : own goal contains at least 1 own action variable [default: 0] (only for randomBG)
- -u : the probability that a random agent is a (anti-)partner (only for projectBG)
- -v : the number of projects [default: 1] (only for projectBG)
- -z : add costs and constraints [default: 0] (only for projectBG)
compile with:
g++ bg2aspnormal.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc timer.cc command_line_options.cc -O3 -o bg2aspnormal
Warning: this centralized method does not support BGs with costs or constraints.
Please note that this program uses Smodels and Lparse. To use it, you also need to install these, freely available at http://www.tcs.hut.fi/Software/smodels/.
- bg2naive
This is naive heuristic method to compute PNEs or core elements of BGs: it randomly picks an outcome and checks whether the outcome is a solution. Benchmarks are ran with a BG generator (either 'generateprojectbg', 'generatebgforasp', 'generatebgneglit2', 'generatedagbg' or 'generatewsnbg', depending on the specified parameters). It has multiple parameters:
Algorithm related options:
- -f : the file specifying the Boolean game
- -i : the maximum number of iterations [default: 10000]
- -s : the ms before the algorithm is abrupted [default: no timeout]
- -r : the number of runs per Boolean game [default: 1]
- -e : search for PNEs (as opposed to core elements) [default: 1]
- -b : the number of benchmark runs (1 run per game) (overrides -f)
- -a : the number of agents
- -t : the number of actions per agent (only for randomBG)
- -m : the maximum number of operators per goal (only for randomBG)
- -c : the probability of conjunctions (only for randomBG)
- -d : the probability of disjunctions (only for dagBG)
- -j : the probability that agent i depends on agent j (j ≥ i) (only for DAGBG)
- -g : the probability of negations (only for randomBG)
- -o : own goal contains at least 1 own action variable [default: 0] (only for randomBG)
- -u : the probability that a random agent is a (anti-)partner (only for projectBG)
- -v : the number of projects [default: 1] (only for projectBG)
- -z : add costs and constraints [default: 0] (only for projectBG)
compile with:
g++ bg2naive.cc utilities.cc atom.cc proposition.cc asphelper.cc propparser.cc bgstructure.cc bgparser.cc naive.cc timer.cc command_line_options.cc -O3 -o bg2naive
This method supports BGs with costs or constraints.
- bg2tbrs
This is technique to compute PNEs of BGs, based on an tabu best-response search (TBRS) for normal form games (2005). It can be used in a centralized and a decentralized way. In addition to PNEs, the centralized variant can compute core elements. Benchmarks are ran with a BG generator (either 'generateprojectbg', 'generatebgforasp', 'generatebgneglit2', 'generatedagbg' or 'generatewsnbg', depending on the specified parameters). It has multiple parameters:
Algorithm related options:
- -f : the file specifying the Boolean game
- -l : the maximum length of the tabu-list [default: 10]
- -i : the maximum number of iterations [default: 100000]
- -s : the ms before the algorithm is abrupted [default: no timeout]
- -r : the number of runs per Boolean game [default: 1]
- -e : search for PNEs (as opposed to core elements) [default: 1]
- -x : use the centralized variant (as opposed to the decentralized variant) [default: 1]
- -b : the number of benchmark runs (1 run per game) (overrides -f)
- -a : the number of agents
- -t : the number of actions per agent (only for randomBG)
- -m : the maximum number of operators per goal (only for randomBG)
- -c : the probability of conjunctions (only for randomBG)
- -d : the probability of disjunctions (only for dagBG)
- -j : the probability that agent i depends on agent j (j ≥ i) (only for DAGBG)
- -g : the probability of negations (only for randomBG)
- -o : own goal contains at least 1 own action variable [default: 0] (only for randomBG)
- -u : the probability that a random agent is a (anti-)partner (only for projectBG)
- -v : the number of projects [default: 1] (only for projectBG)
- -z : add costs and constraints [default: 0] (only for projectBG)
- -w : a global solution exists (as opposed to no global solution exists) [default: 0] (only for randomBG)
compile with:
g++ bg2tbrs.cc utilities.cc atom.cc proposition.cc propparser.cc asphelper.cc bgstructure.cc bgparser.cc tbrs.cc timer.cc command_line_options.cc -O3 -o bg2tbrs
This method supports BGs with costs or constraints.
Source Code Files
The source code files for the Boolean games solver can be downloaded as a zip-file below:Boolean Games Solver - source code files [zip]
Data
- The Boolean games used for the experiments in the paper Decentralized computation of Pareto optimal pure Nash equilibria of Boolean games with privacy concerns can be downloaded as a zip-file below:
WSLpS - experimental data [zip]
The BG files are collected in three folders, each corresponding to one of the experiments.
For the first and third experiment, the generator 'generatebg' was used, for the second 'generatebgneglit2'.
The program 'bg2wslps' was used to run the experiments.
- The Boolean games used for the experiments in the paper Using Answer Set Programming for Solving Boolean Games can be downloaded as a zip-file below:
Boolean Games Solver - experimental data [zip]
The zip-file contains 2 folders:- exp_projectBG: the project Boolean games generated with 'generateprojectbg'.
The game files are divided in 3 subfolders, each corresponding to the number of projects.
The filenames are "projectBG_{#projects}proj_{#agents}agents_{seed}.txt".
- exp_randomBG: the random Boolean games generated with 'generatebgforasp'.
The game files are divided in 3 subfolders, each corresponding to a parameterset: 2 action variables per agent and a maximum of 3 binary operators per goal, 2 action variables per agent and a maximum of 6 binary operators per goal, and 4 action variables per agent and a maximum of 6 binary operators per goal.
The filenames are "randomBG_{#action variables}act_{#binary operators}op_{#agents}agents_{seed}.txt".
- exp_projectBG: the project Boolean games generated with 'generateprojectbg'.
- The Boolean games used for the experiments in the paper Exact and Heuristic Methods for Solving Boolean Games can be downloaded as a zip-file below:
Boolean Games Solver - experimental data [zip]
The zip-file contains 5 folders:- exp_dagBG: the DAG Boolean games generated with 'generatedagbg'.
The game files are divided in 5 subfolders, each corresponding to a parameterset:- 2 action variables per agent and a maximum of 4 operators per goal;
- 2 action variables per agent and a maximum of 7 operators per goal;
- 4 action variables per agent and a maximum of 7 operators per goal;
- 6 action variables per agent and a maximum of 10 operators per goal; and
- 8 action variables per agent and a maximum of 10 operators per goal;
The filenames are "dagBG_{#action variables}act_{#binary operators}op_{#agents}agents_{seed}.txt".
- exp_projectBG: the project Boolean games generated with 'generateprojectbg'.
The game files are divided in 20 subfolders, each corresponding to a specific set of parameters.
The filenames of games without costs and constraints are
"projectBG_{partnerprobability without decimal symbol}partnprob_{#projects}proj_{#agents}agents_{seed}.txt".
The filenames of games with costs and constraints are
"projectBG_{partnerprobability without decimal symbol}partnprob_{#projects}proj_cc_{#agents}agents_{seed}.txt".
- exp_randomBG: the random Boolean games generated with 'generatebgforasp'.
The game files are divided in 5 subfolders, each corresponding to a parameterset:- 2 action variables per agent and a maximum of 3 operators per goal;
- 2 action variables per agent and a maximum of 6 operators per goal;
- 4 action variables per agent and a maximum of 6 operators per goal;
- 6 action variables per agent and a maximum of 10 operators per goal; and
- 8 action variables per agent and a maximum of 10 operators per goal;
The filenames are "randomBG_{#action variables}act_{#binary operators}op_{#agents}agents_{seed}.txt".
- exp_randomBG_glob: the random Boolean games generated with 'generatebgneglit2'.
The filenames of games for which the number of binary operators is fixed are
"randomBG_glob_{#action variables}act_{#conjunctions}conj_{#disjunctions}disj_fixed_{#agents}agents_{seed}.txt".
The filenames of games for which the number of binary operators has an upperbound are
"randomBG_glob_{#action variables}act_{#conjunctions}conj_{#disjunctions}disj_max_{probability of atom being negated, without decimal symbol}neg_{#agents}agents_{seed}.txt".
- exp_WSNBG: the wireless sensor network Boolean games generated with 'generatewsnbg'.
The filenames are "WSNBG_{topology}_{#agents}agents_{seed}.txt".
- exp_dagBG: the DAG Boolean games generated with 'generatedagbg'.
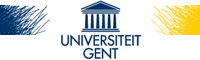